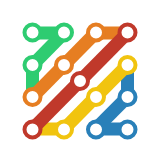 |
FFmpegKit Linux API
4.5.1
|
Go to the documentation of this file.
44 #ifndef FFTOOLS_FFMPEG_H
45 #define FFTOOLS_FFMPEG_H
53 #include "libavformat/avformat.h"
54 #include "libavformat/avio.h"
56 #include "libavcodec/avcodec.h"
57 #include "libavcodec/bsf.h"
59 #include "libavfilter/avfilter.h"
61 #include "libavutil/avutil.h"
62 #include "libavutil/dict.h"
63 #include "libavutil/eval.h"
64 #include "libavutil/fifo.h"
65 #include "libavutil/hwcontext.h"
66 #include "libavutil/pixfmt.h"
67 #include "libavutil/rational.h"
68 #include "libavutil/thread.h"
69 #include "libavutil/threadmessage.h"
71 #include "libswresample/swresample.h"
74 #define VSYNC_PASSTHROUGH 0
77 #define VSYNC_VSCFR 0xfe
78 #define VSYNC_DROP 0xff
80 #define MAX_STREAMS 1024
330 #define DECODING_FOR_OST 1
331 #define DECODING_FOR_FILTER 2
451 AVThreadMessageQueue *in_thread_queue;
455 int thread_queue_size;
468 #define ABORT_ON_FLAG_EMPTY_OUTPUT (1 << 0)
469 #define ABORT_ON_FLAG_EMPTY_OUTPUT_STREAM (1 << 1)
664 extern __thread
const AVIOInterruptCB
int_cb;
668 extern __thread
char *qsv_device;
685 enum AVPixelFormat
choose_pixel_fmt(AVStream *st, AVCodecContext *avctx,
const AVCodec *codec,
enum AVPixelFormat target);
715 void set_report_callback(
void (*callback)(
int,
float,
float, int64_t,
int,
double,
double));
719 int opt_map(
void *optctx,
const char *opt,
const char *arg);
723 int opt_progress(
void *optctx,
const char *opt,
const char *arg);
724 int opt_target(
void *optctx,
const char *opt,
const char *arg);
725 int opt_vsync(
void *optctx,
const char *opt,
const char *arg);
726 int opt_abort_on(
void *optctx,
const char *opt,
const char *arg);
728 int opt_qscale(
void *optctx,
const char *opt,
const char *arg);
729 int opt_profile(
void *optctx,
const char *opt,
const char *arg);
732 int opt_attach(
void *optctx,
const char *opt,
const char *arg);
735 int opt_sameq(
void *optctx,
const char *opt,
const char *arg);
736 int opt_timecode(
void *optctx,
const char *opt,
const char *arg);
739 int opt_vstats(
void *optctx,
const char *opt,
const char *arg);
741 int opt_old2new(
void *optctx,
const char *opt,
const char *arg);
742 int opt_streamid(
void *optctx,
const char *opt,
const char *arg);
743 int opt_bitrate(
void *optctx,
const char *opt,
const char *arg);
744 int show_hwaccels(
void *optctx,
const char *opt,
const char *arg);
750 int opt_preset(
void *optctx,
const char *opt,
const char *arg);
755 int opt_sdp_file(
void *optctx,
const char *opt,
const char *arg);
756 int opt_data_codec(
void *optctx,
const char *opt,
const char *arg);
768 const AVCodec *
find_codec_or_die(
const char *name,
enum AVMediaType type,
int encoder);
771 int get_preset_file_2(
const char *preset_name,
const char *codec_name, AVIOContext **s);
SpecifierOpt * disposition
SpecifierOpt * hwaccel_output_formats
double rotate_override_value
AVCodecParameters * ref_par
const char ** attachments
char * filters
filtergraph associated to the -filter option
AVFifoBuffer * muxing_queue
int nb_audio_channel_maps
SpecifierOpt * enc_time_bases
SpecifierOpt * chroma_intra_matrices
SpecifierOpt * dump_attachment
SpecifierOpt * sample_fmts
SpecifierOpt * filter_scripts
SpecifierOpt * top_field_first
size_t muxing_queue_data_threshold
int audio_channels_mapped
const char * attachment_filename
SpecifierOpt * rc_overrides
int metadata_chapters_manual
SpecifierOpt * frame_rates
AVRational max_frame_rate
SpecifierOpt * forced_key_frames
SpecifierOpt * guess_layout_max
int max_muxing_queue_size
SpecifierOpt * frame_aspect_ratios
double forced_keyframes_expr_const_values[FKF_NB]
struct FilterGraph * graph
int nb_copy_initial_nonkeyframes
AVExpr * forced_keyframes_pexpr
SpecifierOpt * max_frames
enum AVPixelFormat pix_fmt
SpecifierOpt * max_muxing_queue_size
SpecifierOpt * audio_sample_rate
int64_t recording_time
desired length of the resulting file in microseconds == AV_TIME_BASE units
int metadata_global_manual
AudioChannelMap * audio_channel_maps
int64_t forced_kf_ref_pts
SpecifierOpt * passlogfiles
SpecifierOpt * hwaccel_devices
SpecifierOpt * frame_pix_fmts
AVDictionary * resample_opts
SpecifierOpt * bitstream_filters
SpecifierOpt * autorotate
SpecifierOpt * inter_matrices
SpecifierOpt * frame_sizes
struct InputStream * sync_ist
SpecifierOpt * metadata_map
int nb_frame_aspect_ratios
int nb_max_muxing_queue_size
SpecifierOpt * time_bases
SpecifierOpt * intra_matrices
SpecifierOpt * copy_initial_nonkeyframes
SpecifierOpt * copy_prior_start
SpecifierOpt * max_frame_rates
SpecifierOpt * codec_names
SpecifierOpt * fix_sub_duration
SpecifierOpt * reinit_filters
char * filters_script
filtergraph script associated to the -filter_script option
size_t muxing_queue_data_size
int(* init)(AVCodecContext *s)
SpecifierOpt * canvas_sizes
AVRational frame_aspect_ratio
int metadata_streams_manual
SpecifierOpt * muxing_queue_data_threshold
SpecifierOpt * audio_channels
int64_t start_time
start time in microseconds == AV_TIME_BASE units
int nb_hwaccel_output_formats
int copy_initial_nonkeyframes
int nb_muxing_queue_data_threshold
AVDictionary * encoder_opts
int nb_chroma_intra_matrices
struct OutputStream * ost
uint64_t * channel_layouts
SpecifierOpt * codec_tags